for of usage
//2.for of usage
let productDetails = [
{
product_Id: 1,
product_Name: "LG Monitor",
price: "55k",
rating: "super",
},
{
product_Id: 2,
product_Name: "LG Curve Monitor",
price: "45k",
rating: "Average",
},
];
for (let obj of productDetails) {
console.log(JSON.stringify(obj));
}
/*
Basically used to send data to backend server
{"product_Id":1,"product_Name":"LG Monitor","price":"55k","rating":"super"}
{"product_Id":2,"product_Name":"LG Curve Monitor","price":"45k","rating":"Average"}
*/
Basically used to send data to backend server
JSON.PARSE USAGE IN LOCAL STORAGE
let employee = {
name: "Anurag",
code: "empCode-123",
};
const obj = JSON.stringify(employee);
console.log(obj); //{"name":"Anurag","code":"empCode-123"} -->in string
localStorage.setItem("key", obj);
//WRONG KEY
console.log(JSON.parse(localStorage.getItem("key1"))); //null
//CORRECT KEY
console.log(JSON.parse(localStorage.getItem("key"))); //{name: 'Anurag', code: 'empCode-123'}
//CORRECT KEY WITOUT PARSE
console.log(localStorage.getItem("key")); //{name: 'Anurag', code: 'empCode-123'} -->in string...
localStorage.setItem("key", obj);
JSON.parse(localStorage.getItem("key"))
JSON.parse(localStorage.getItem("key"))
Change the background color
//HTML part
//Javascript part
const changeColor = (color) => {
document.body.style.background = color;
};
document.querySelector("#blue").addEventListener("click", () => changeColor("blue"));
document.querySelector("#green").addEventListener("click", () => changeColor("green"));
document.querySelector("#yellow").addEventListener("click", () => changeColor("yellow"));
Password Validity Question
function checkPassword(password) {
let onelowercaseLetter = /(?=.+[a-z])/;
let oneUppercaseLetter = /(?=.+[A-Z])/;
let oneDigit = /(?=.+[0-9])/;
let oneSpecialCharacter = /(?=.+[!@#$%^&*])/;
let minEightDigit = /(?=.{8,})/;
let isValid =
onelowercaseLetter.test(password) &&
oneUppercaseLetter.test(password) &&
oneDigit.test(password) &&
oneSpecialCharacter.test(password) &&
minEightDigit.test(password);
return isValid ? "passoword is valid" : "password is invalid";
}
console.log(checkPassword("aZ#1333ddd3"));
console.log(checkPassword("anurag"));
JSON.stringify certain properties
const address = { city: "Rayagada", state: "Odisha" };
const employee = { name: "Anurag", gender: "Male", ...address };
console.log(employee); //{name: 'Anurag', gender: 'Male', city: 'Rayagada', state: 'Odisha'}
//{"name":"Anurag","gender":"Male","state":"Odisha"}
//it only strigified --> name , gender , state
console.log(JSON.stringify(employee, ["name", "gender", "state"]));
Deep cloning
Attempt 1 - Direct Assignment
⦿ Age is getting updated in both source and destination, when we change the destination object(which is wrong)
//********** Deep cloning  **************
var cricketer = {
 name: "Virat",
 team: "India",
 age: 32,
 colleagues: {
  name: "Rohit",
 },
 performance: function (type) {
  return `${this.name} is ${type} player`;
 },
};
var copyCricketer = cricketer;
copyCricketer.age = 42;
console.log(copyCricketer);
console.log(cricketer);

Attempt 2 - JSON.stringify
⦿ Age is updating in destination withou affecting the source which is correct
⦿ Function is missing in destination which is wrong
⦿ Function is missing in destination which is wrong
var copyCricketer1 = JSON.parse(JSON.stringify(cricketer));
copyCricketer1.age = 45;
console.log(copyCricketer1);
console.log(cricketer);
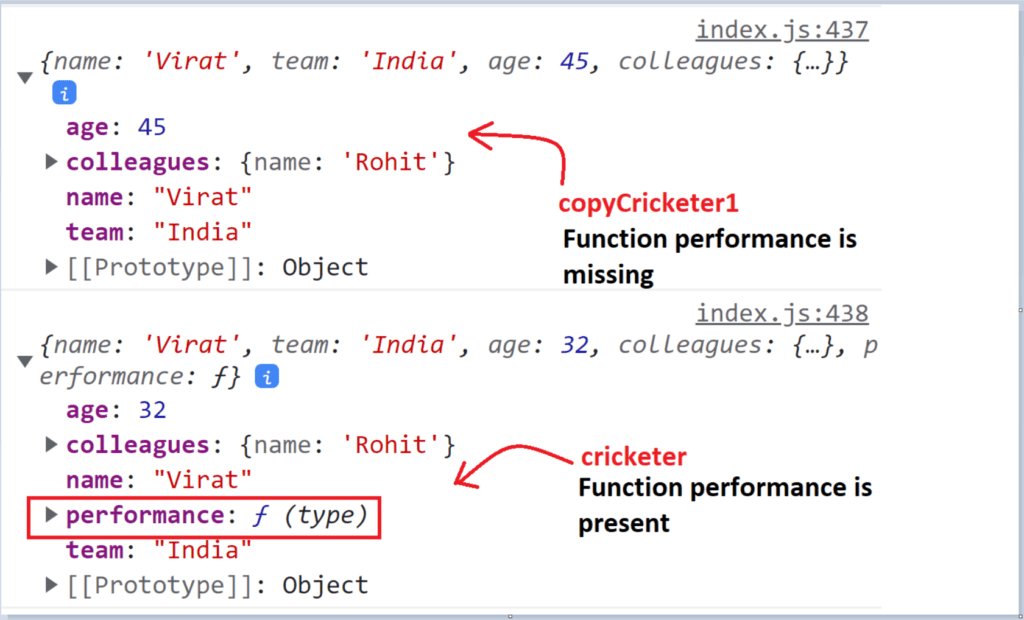
Attempt 3 - Object.Assign
⦿ We changed Rahane in destination nested object. But
Rahane is copied in both source and destination both which is wrong.
⦿ Age is updating properly in destination without affecting source
⦿ so nested object is getting affected in both source and destination
⦿ Age is updating properly in destination without affecting source
⦿ so nested object is getting affected in both source and destination
var copyCricketer2 = Object.assign({}, cricketer);
copyCricketer2.colleagues.name = "Rahane";
copyCricketer2.age = 34;
console.log(copyCricketer2);
console.log(cricketer);
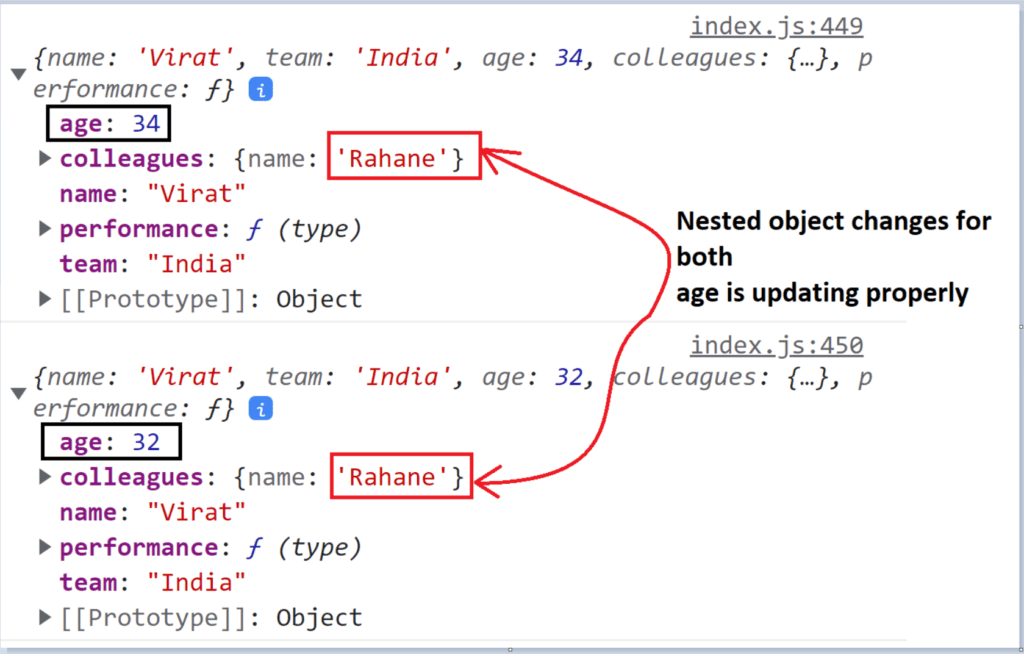
Attempt 4 - spread operator
⦿ Behaves similarly as Objecgt.assign
⦿ Nested objects are copied in both source and destination
⦿ Nested objects are copied in both source and destination
let spreadCricketerCopy = { ...cricketer };
spreadCricketerCopy.colleagues.name = "Rahane";
spreadCricketerCopy.age = 34;
console.log(spreadCricketerCopy);
console.log(cricketer);
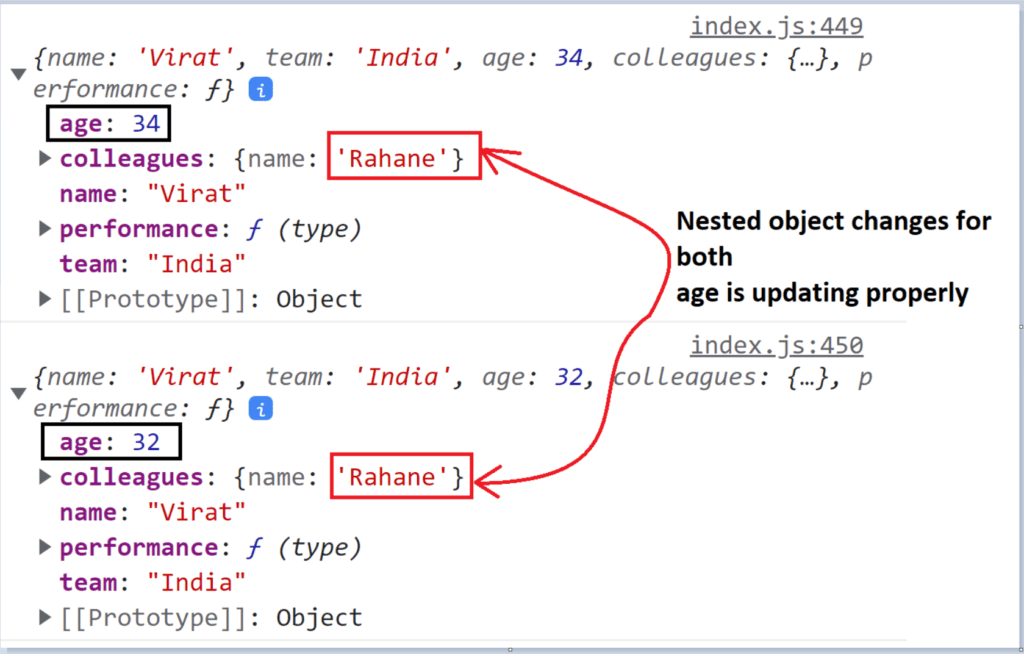
Attempt 5 - use loadash (_.cloneDeep)
It copies properly without any side effects
let finalDeepCopy = _.cloneDeep(cricketer);
finalDeepCopy.colleagues.name = "Rahane";
console.log(finalDeepCopy);
console.log(cricketer);
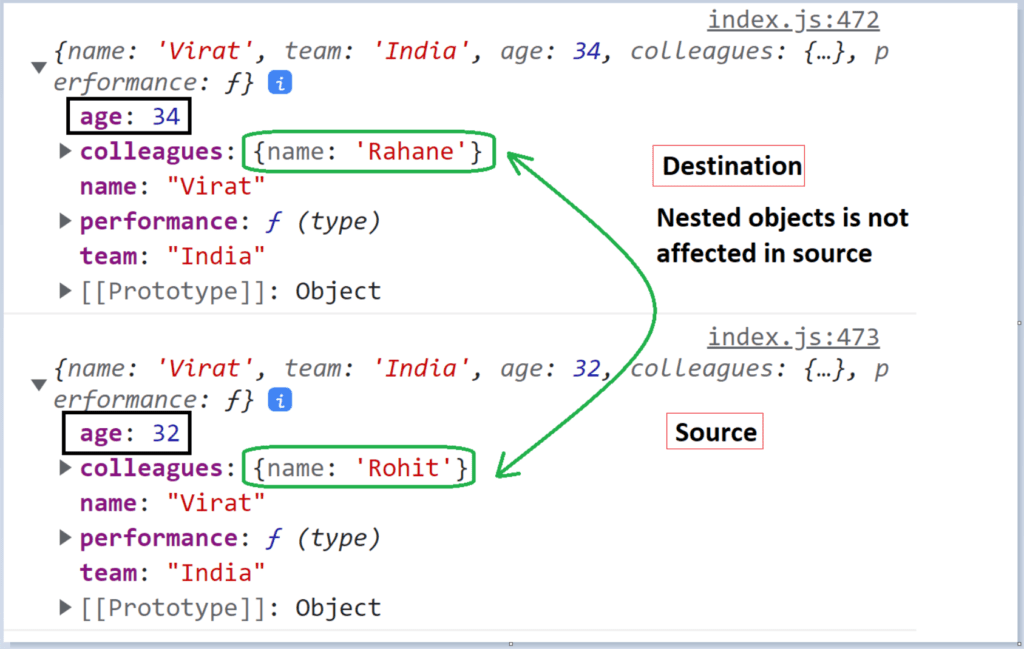
Generate Random number
//Generate Random Number
Math.random(); //gives you decimal between 0 and 1
//I want to generate random number between 1 and 100
Math.ceil(Math.random()) * 100;
//I want to generate random number between 1 and 10
Math.ceil(Math.random()) * 10;
//I want to generate random number between 20 and 30
Math.ceil(Math.random()) * 10 + 20;
//I want to generate random number between 20 and 50
Math.ceil(Math.random() * (50 - 20)) + 20;
create a private variable in Javascript
const accessPrivateKey = () => {
let privateKey = "Private Key";
return function () {
return privateKey;
};
};
let getPrivateKey = accessPrivateKey();
console.log(getPrivateKey()); //Private Key
console.log(accessPrivateKey());
//
// Æ’ () {
// return privateKey;
// }