Key Points
- Do npm init
- Generate the package.json
- Install faker from below link
- https://www.npmjs.com/package/@faker-js/faker
- npm install –save-dev @faker-js/faker
- “filldata”: “node app.js > db.json“
- node app.js will generte the employees array
- export const Users = {Â employees: [],};
- Â console.log(JSON.stringify(Users));
- This will generate {“employees”:[  { “id”: ” ….},  { “id”: ” ….},]
}
- This will generate {
- Now all we need to do is to push to db.json
- “filldata”: “node app.js > db.json”,
- This is written in package.json
- This will push data to db.json from above generated employees object
- db.json works only on object. So make sure you dont do any mistake of keeping array in db.json
- That is the reason if you see users is object , that has employees which we populatedexport const Users = {Â employees: [],};
- That is the reason if you see users is object , that has employees which we populated
- “populateAndLoad“: “npm run filldata &&Â json-server –watch db.json“
- npm run filldata :- This will do the filldata – which pushes data from app.js to db.json
- json-server –watch db.json :-Â This will run the json-server at default port 3000
- But you can specifially run at specific port
- json-server –watch db.json –port 3004Â
- Do npm run populateAndLoad to load the db.json and start loading the data
- Use postman to put, post, delete , get. This will update the db.json.
app.js
import { faker } from "@faker-js/faker";
export const Users = {
employees: [],
};
export function createRandomUser() {
return {
id: faker.datatype.uuid(),
employeeName: faker.internet.userName(),
employeeEmail: faker.internet.email(),
empDob: faker.date.birthdate(),
};
}
Array.from({ length: 10 }).forEach(() => {
Users.employees.push(createRandomUser());
});
console.log(JSON.stringify(Users));
db.json
{
"employees": [
{ "id": "de94ca2b-a9bf-4db6-911f-3bdf9fb35ad5", "employeeName": "Craig13", "employeeEmail": "Cayla_Mills@hotmail.com", "empDob": "1957-07-17T12:28:45.876Z" },
{ "id": "ca49a19e-8691-4600-aad2-a5659240fddc", "employeeName": "Claudie13", "employeeEmail": "Fannie_Schmidt@hotmail.com", "empDob": "2004-10-01T15:56:07.127Z" },
{ "id": "baf97409-3094-4bd4-b974-89fdc7332f44", "employeeName": "Rosanna93", "employeeEmail": "Mckayla56@yahoo.com", "empDob": "1944-01-08T12:33:29.036Z" },
{ "id": "57052a8e-b29b-4210-8bca-b1896de0ce8f", "employeeName": "Cassidy.Gottlieb", "employeeEmail": "Elyse.Champlin65@yahoo.com", "empDob": "1954-05-23T08:46:05.695Z" },
{ "id": "46ce29c5-44a4-42aa-8295-4c4379ee5e11", "employeeName": "Jovanny79", "employeeEmail": "Bettye_Windler@yahoo.com", "empDob": "1982-05-30T10:22:16.942Z" },
{ "id": "4f49401d-d117-4ac1-a4f2-32f5eb29a706", "employeeName": "Irma_Schulist", "employeeEmail": "Kian.Hilpert45@gmail.com", "empDob": "1987-09-02T17:01:03.009Z" },
{ "id": "8b746f0e-5cbe-46a6-81b7-f740f3f1c75a", "employeeName": "Enola71", "employeeEmail": "Allan35@hotmail.com", "empDob": "1956-07-04T01:12:18.988Z" },
{ "id": "a789a500-05e0-4a56-86e9-4a871f79b5b9", "employeeName": "Torrey7", "employeeEmail": "Sandrine81@hotmail.com", "empDob": "1978-08-23T10:23:30.863Z" },
{ "id": "c2a24219-a60c-444f-b487-cd372534737f", "employeeName": "Morton_Willms14", "employeeEmail": "Tremayne.Hoppe87@hotmail.com", "empDob": "1952-11-14T07:56:29.745Z" },
{ "id": "ca7a391b-daa6-4406-8e35-dac24147208c", "employeeName": "Hudson_Leuschke", "employeeEmail": "Claud.Franecki36@hotmail.com", "empDob": "2002-02-13T15:33:56.680Z" }
]
}
package.json
{
"name": "fake",
"version": "1.0.0",
"description": "",
"main": "app1.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"filldata": "node app.js > db.json",
"populateAndLoad": "npm run filldata && json-server --watch db.json --port 3004"
},
"author": "",
"license": "ISC",
"dependencies": {
"@faker-js/faker": "^7.6.0",
"faker": "^6.6.6"
}
}
OUTPUT
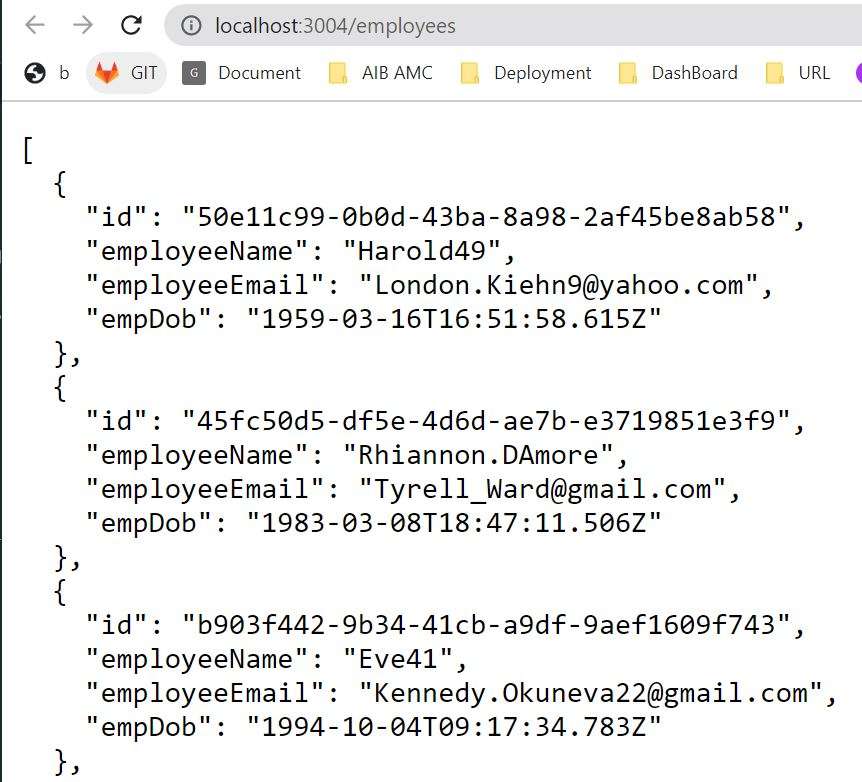
GIT URL