BASICS
- Built by facebook in 2013
- By far the most popular among Angular,Vue, Svelte
- Its all about components. Indpendent chunks of user interface.
- Components are reusable.
- Uses Virtual DOM
- Virtual views in memory –> Generate HTML using Javascript
- No HTML template language
- Tree reconcilation
- Be good at Javascript, everything will be easy
INSTALLATION
- Install node.js – node js is cross platform Javascript runtime environment which allows us to build fast scalable network applications.
- Install the stable version.
- Check the node version.
- node –v
SOME POINTS
- Virtual DOM
- Just Javascript
- Battle tested
- Declarative language
- We describe user interfaces with React and tell it what we want, and not how to do it. React will take care of the how and translate our declarative descriptions , which we write in react language to actual user interfaces in the browser.
- Frameworks- disadvantages
- Do things in a certain way
- hard to deviate
- Framework is large and full of features – hard to customize
JSX IS NOT HTML
- This is JSX
- return <div>Arrow function !!</div>;
- Use this below link and play around.
- https://jscomplete.com/playground
- Playground is using a special compiler called Babel – to convert JSX into React API calls.
- return React.createElement(“div”, null, “Arrow Function!!!”);
- First is the element to be created
- Second is the attribute name – here we dont have any attribute so null
- Child of div elements , here its only string “Arrow Function!!!”
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
const App = () => {
return Arrow function !!;
};
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
);
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
const App = () => {
return React.createElement("div", null, "Arrow Function!!!");
};
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
);
USEFUL EXTENSION TO INSTALL
- Auto Rename tag
- indent-rainbow
- Highlight Matching Tag
- Prettier
- format on save
- format on paste
- Default formatter dropdown (Prettier – code formatter)
- Install the extension ES7 Snippets in visual studio code
- racfe – arrow function with export
- rfce – regular function
- Install React Dev tool extension – this is for browser extension not vscode extension.
- h3#myId.myclass —> <h3 id=”myId” className=”myclass”></h3>
- Go to File > Preferences > Settings
- “emmet.includeLanguages”: {“javascript”: “javascriptreact”}
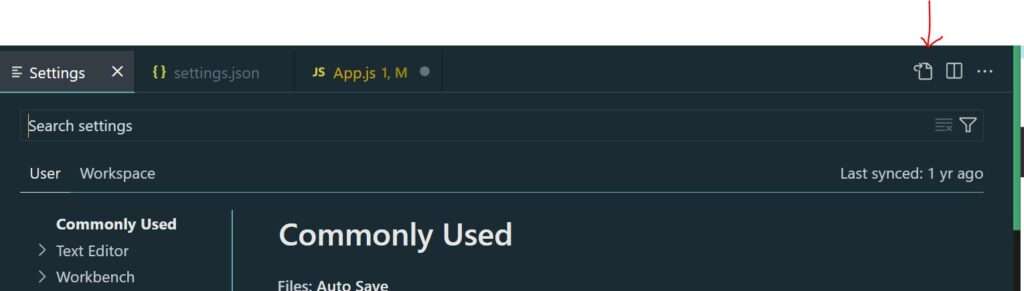
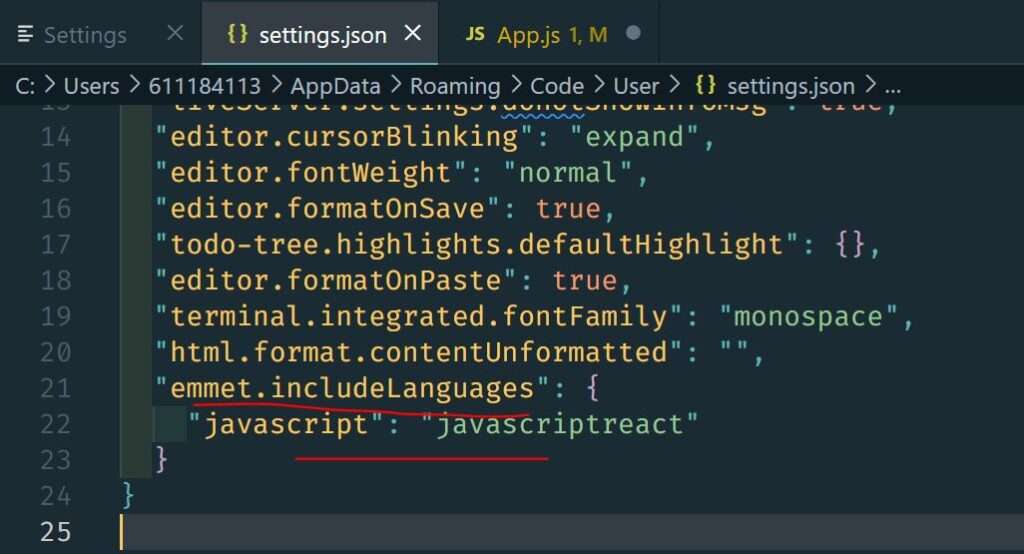