Basics
- Dont miss out the [] the second parameter
- If you miss it , it will be infinite render
- useEffect will call –> getLatestPosts()…it will SetPosts.. SetPosts will change posts. Because post is chaning it will call useEffect again. It renders in loop.
- useEffect(() => {getLatestPosts();}, []);
Program
FetchBlogPost.js
import React, { useEffect, useState } from "react";
import Table from "react-bootstrap/Table";
import "bootstrap/dist/css/bootstrap.min.css";
const myBlogPostUrl = "https://clientsidecoding.com/wp-json/wp/v2/posts";
const FetchBlogPost = () => {
const [posts, SetPosts] = useState([]);
const getLatestPosts = async () => {
const response = await fetch(myBlogPostUrl);
const blogPosts = await response.json();
SetPosts(blogPosts);
};
useEffect(() => {
getLatestPosts();
}, []);
return (
<>
Post
Link
{posts.map((data) => {
return (
{data.slug}
{data.link}
);
})}
>
);
};
export default FetchBlogPost;
index.js
import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
import FetchBlogPost from "./FetchBlogPost";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<>
>
);
OUTPUT
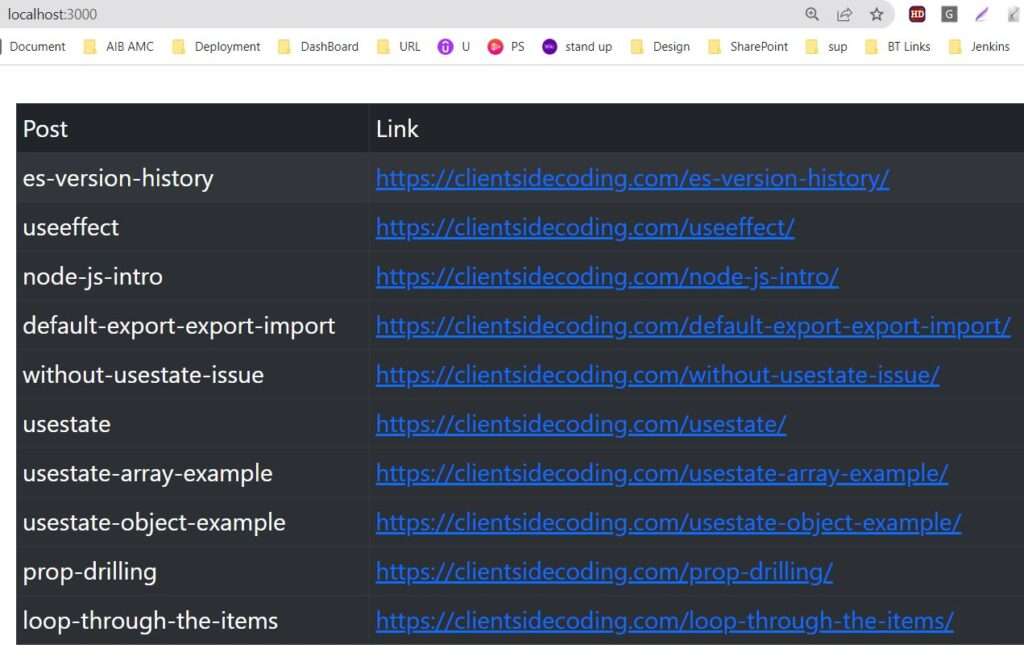
GIT URL