.at(-index)
OLD WAY
//Old Code
let name1 = "anurag";
console.log(name1[name1.length - 3]); //r
let arr1 = [1, 2, 3, 4, 5];
console.log(arr1[arr1.length - 3]); //3
NEW WAY
//New Code
let name2 = "anurag";
console.log(name2.at(-3)); //r
let arr2 = [1, 2, 3, 4, 5];
console.log(arr2.at(-3)); //3
hasOwn()
Object.hasOwn() can be used as a replacement for Object.hasOwnProperty()
OLD WAY
//Old Way
const addressObj = { city: "Imphal" };
console.log(addressObj.hasOwnProperty("city")); // true
console.log(addressObj.hasOwnProperty("street")); // false
NEW WAY
//New Way
const addressObj = { city: "Imphal" };
console.log(Object.hasOwn(addressObj, "city")); // true
console.log(Object.hasOwn(addressObj, "street")); // false
Create object using Object.create(existingobject) - hasOwn vs hasOwnProperty()
const addressObj = { city: "Imphal" };
const addressObj1 = Object.create(addressObj);
console.log(Object.hasOwn(addressObj1, "city")); // false - coz it doesnt have any city property
console.log(addressObj1.hasOwnProperty("city")); //false - coz it doesnt has its own property city
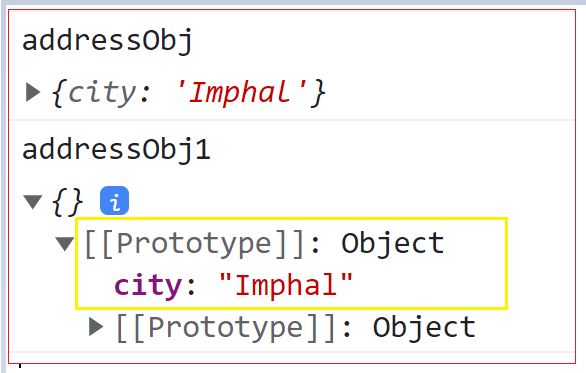
Create object using Object.create(null)-- hasOwn vs hasOwnProperty()
const addressObj2 = Object.create(null);
console.log(Object.hasOwn(addressObj2, "city")); // false - as it doesnt have city property
console.log(addressObj2.hasOwnProperty("city")); //false - it doesnt have any prototype properties ...so nohasOwnProperty so error as per below screenshot
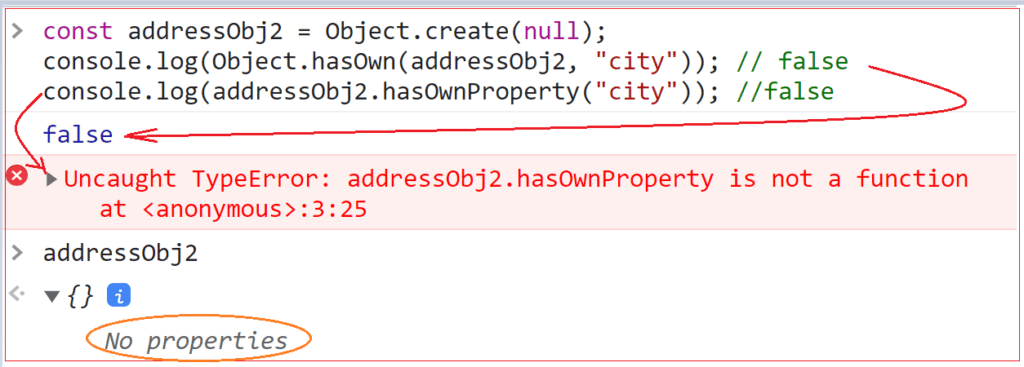
const addressObj3 = Object.create(null);
addressObj3.city='Mumbai';
console.log(Object.hasOwn(addressObj3, "city")); // true -it has city property created in above line
console.log(addressObj3.hasOwnProperty("city")); //false - doesnt have any prototype properties - error
Create object using Object.create(null) and assign property to the new object
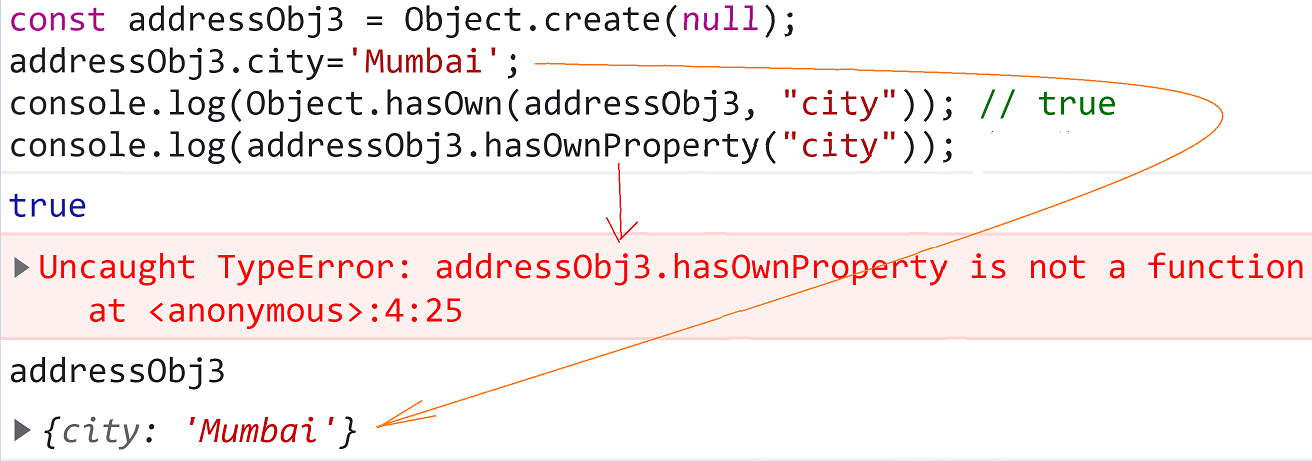
Top Level Await
//Top Level Await
// 1. Old way
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then((response) => response.json())
.then((json) => console.log(json));
// 2. Old way
const fetchTodoData = async () => {
const response = await fetch("https://jsonplaceholder.typicode.com/todos/1");
const data = await response.json();
console.log(data);
};
fetchTodoData();
//New Way - Top Level Await
const response = await fetch("https://jsonplaceholder.typicode.com/todos/1");
const data = await response.json();
console.log(data);
Private fields
OLD WAY
class Calculation {
randomValue = Math.random();
generateValueFiveToTen() {
return Math.floor(5 + (10 - 5) * this.randomValue);
}
}
let obj = new Calculation();
console.log(obj.randomValue); //Able to access randomValue variable of class
console.log(obj.generateValueFiveToTen());//random value between 5 to 9
# BEFORE THE VARIABLE -MAKE VARIABLE PRIVATE
class Calculation {
#randomValue = Math.random();
generateValueFiveToTen() {
return Math.floor(5 + (10 - 5) * this.#randomValue);
}
}
let obj = new Calculation();
console.log(obj.randomValue); //undefined
console.log(obj.generateValueFiveToTen()); //random value between 5 to 9
# BEFORE THE METHOD- MAKE METHOD PRIVATE
class Calculation {
#randomValue = Math.random();
#generateValueFiveToTen() {
return Math.floor(5 + (10 - 5) * this.#randomValue);
}
RandomValue() {
return this.#generateValueFiveToTen();
}
}
let obj = new Calculation();
console.log(obj.randomValue); //undefined
console.log(obj.RandomValue()); //random value between 5 to 9
console.log(obj.generateValueFiveToTen()); //Uncaught TypeError: obj.generateValueFiveToTen is not a function