Basics
- Runs after every re-render
- It takes the callback function
- useEffect(() => {});
- This is not allowed
- Â Â if (true) {Â Â useEffect(() => {});Â }
- Multiple use effect is possible – output in the below screenshot
- Â useEffect(() => {Â Â console.log(“hello”);Â }, []);
Â
 useEffect(() => {  console.log(“hello-world”); }, []);
- Â
Dependency Array
- The second parameter is dependency array
- Â useEffect(() => {Â Â console.log(“hello-world”);Â }, []);
- As it is empty array – it will only render once.
- But if you see in other useEffect it takes counter. On every button click the counter value changes, which re-renders the component. The other useEffect will be called.
- Â useEffect(() => {Â Â console.log(“hello”);Â }, [counter]);
import React, { useEffect, useState } from "react";
const UseEffectBasics = () => {
const [counter, setCounter] = useState(0);
useEffect(() => {
console.log("hello");
}, [counter]);
useEffect(() => {
console.log("hello-world");
}, []);
return (
<>
- {counter}
>
);
};
export default UseEffectBasics;
DATA
- The first hello and hello-world is on initial render
- Then 21 clicks –> 21 hello
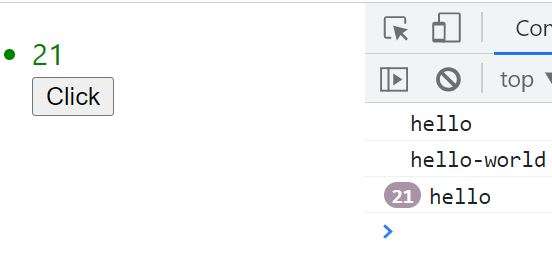