Primitive/Non-Primitive Data Types
key points
⦿ Primitive data type :- string,number,bigint,boolean,undefined,null,symbol
⦿ Non-primitive data type :- object
⦿ Bigint was introduced in ES2020
⦿ Primitive data are those data which are not an object, and has no methods and properties. Also it is immutable.
⦿ Arrays and functions are objects.
⦿ Symbol was introduced in ES2015
⦿ Non-primitive data type :- object
⦿ Bigint was introduced in ES2020
⦿ Primitive data are those data which are not an object, and has no methods and properties. Also it is immutable.
⦿ Arrays and functions are objects.
⦿ Symbol was introduced in ES2015
//*******DataTypes**************
typeof 5 --> number
typeof true --> boolean
typeof 'I am string' --> string
typeof undefined --> undefined
typeof null --> object
typeof Symbol('symbol') --> Symbol
typeof {} --> object
console.log(typeof []); //object (Arrays are objects)
typeof NaN --> Number
//*******Functions**************
const obj = function () {
return 5;
};
obj.hello = "hello";
//Here functions are behaving like objects.you can assign the property
//console.log(obj.hello); // output is hello.
undefined vs null
key points
⦿ undefined == with null returns true
⦿ undefined === with null returns false
⦿ undefined === with null returns false
//****************************************
console.log(`%c ${undefined === null}`, "color:red;font-size:20px");//false
console.log(`%c ${undefined == null}`, "color:red;font-size:20px"); //true
Memory leak
key points
⦿ Runs into infinite loop
⦿ Browser crashes. Kindly see the screenshot below
⦿ Browser crashes. Kindly see the screenshot below
//********************************
let array= [];
for (let i= 15; i > 1; i++) {
array.push(i);
}
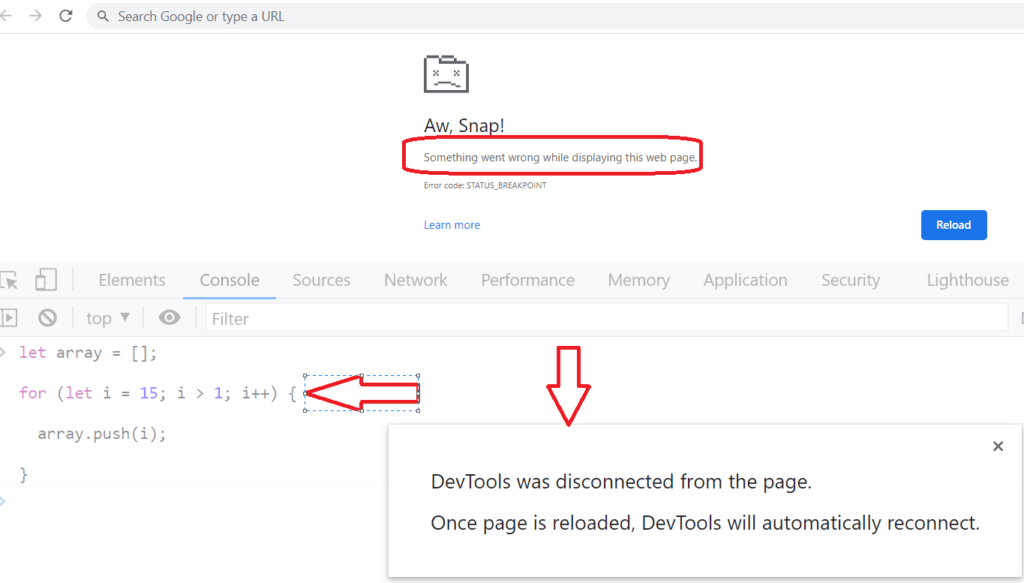
Remove Duplicates in an array
key points
⦿ Arrays and functions are objects.
⦿ symbol was introduced in ES2015
⦿ symbol was introduced in ES2015
//**Remove Duplicate **
const num1 = [1, 2, 3, 4, 5];
const num2 = [2, 3, 6, 7, 8];
let removeDuplicate = [...new Set([...num1, ...num2])];
console.log(`%c ${removeDuplicate}`, "color:red;font-size:20px");
output:- 1,2,3,4,5,6,7,8
// [... new Set(array)
assign values to variable shortcut
//************Assignment**********************
let a, b, c;
//old way
// a = 1;
// b = 2;
// c = 3;
//new way as below
[a, b, c] = [1, 2, 3];
console.log(`%c ${a} ${b} ${c}`, "color:red;font-size:20px");
//output- 1,2,3
Javascript is a dynamic language
key points
⦿ you can assign any data type in javascript, so it is dynamic.
⦿ In the below example, the last value gets updated
⦿ In the below example, the last value gets updated
var a = "anurag";
a = 123;
a = true;
console.log(`%c ${a}`, "color:red;font-size:20px");
//output:- true
var let const - redeclarable/updatable
key points
⦿ var is redeclarable and updatable.
⦿ let cannot be redeclared but updatable.
⦿ const is neither redeclarable nor updatable
⦿ let cannot be redeclared but updatable.
⦿ const is neither redeclarable nor updatable
//****************var************************
var test = "test";
var test = "test changed";
console.log(test);
output:- test changed
//****************let************************
let test = "test";
//below redeclaration is not allowed as let is already declared
let test = "can I redeclare ??";
Deep cloning
Attempt 1 - Direct Assignment
⦿ Age is getting updated in both source and destination, when we change the destination object(which is wrong)
//********** Deep cloning **************
var cricketer = {
name: "Virat",
team: "India",
age: 32,
colleagues: {
name: "Rohit",
},
performance: function (type) {
return `${this.name} is ${type} player`;
},
};
var copyCricketer = cricketer;
copyCricketer.age = 42;
console.log(copyCricketer);
console.log(cricketer);

Attempt 2 - JSON.stringify
⦿ Age is updating in destination withou affecting the source which is correct
⦿ Function is missing in destination which is wrong
⦿ Function is missing in destination which is wrong
var copyCricketer1 = JSON.parse(JSON.stringify(cricketer));
copyCricketer1.age = 45;
console.log(copyCricketer1);
console.log(cricketer);
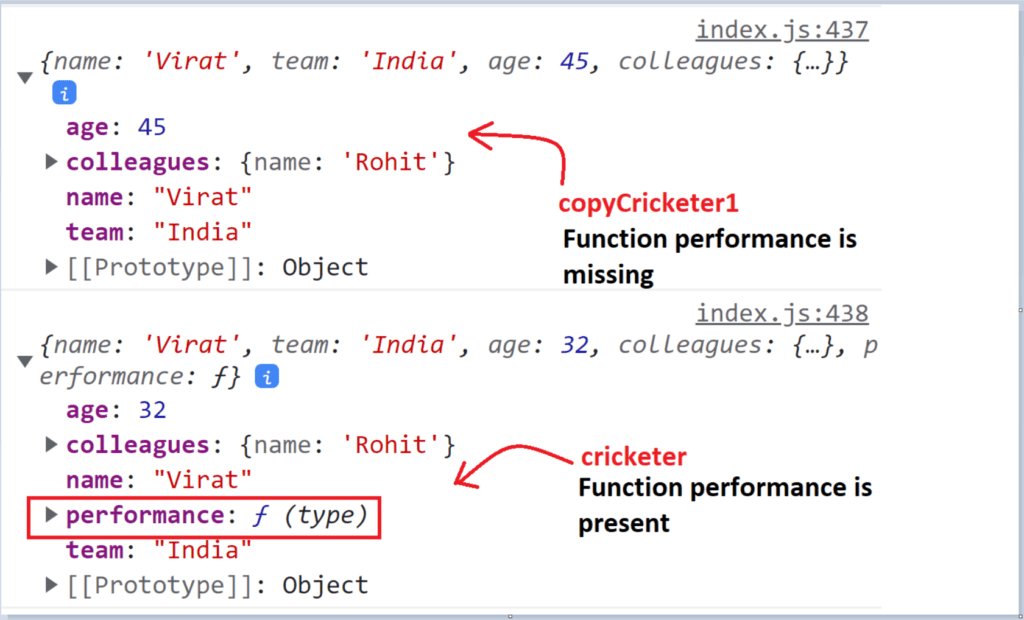
Attempt 3 - Object.Assign
⦿ We changed Rahane in destination nested object. But
Rahane is copied in both source and destination both which is wrong.
⦿ Age is updating properly in destination without affecting source
⦿ so nested object is getting affected in both source and destination
⦿ Age is updating properly in destination without affecting source
⦿ so nested object is getting affected in both source and destination
var copyCricketer2 = Object.assign({}, cricketer);
copyCricketer2.colleagues.name = "Rahane";
copyCricketer2.age = 34;
console.log(copyCricketer2);
console.log(cricketer);
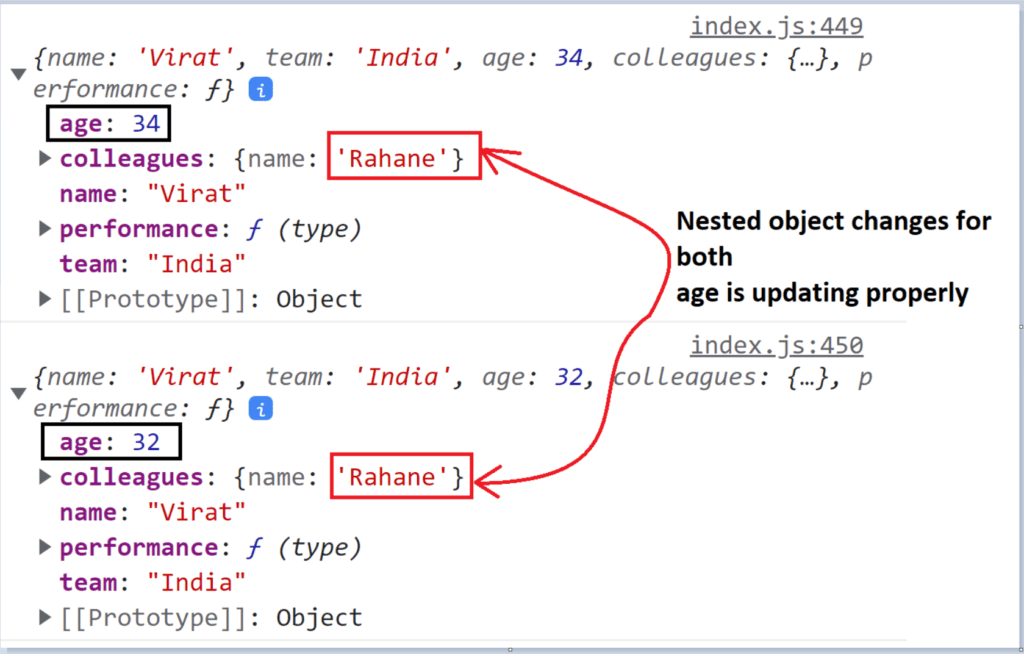
Attempt 4 - spread operator
⦿ Behaves similarly as Objecgt.assign
⦿ Nested objects are copied in both source and destination
⦿ Nested objects are copied in both source and destination
let spreadCricketerCopy = { ...cricketer };
spreadCricketerCopy.colleagues.name = "Rahane";
spreadCricketerCopy.age = 34;
console.log(spreadCricketerCopy);
console.log(cricketer);
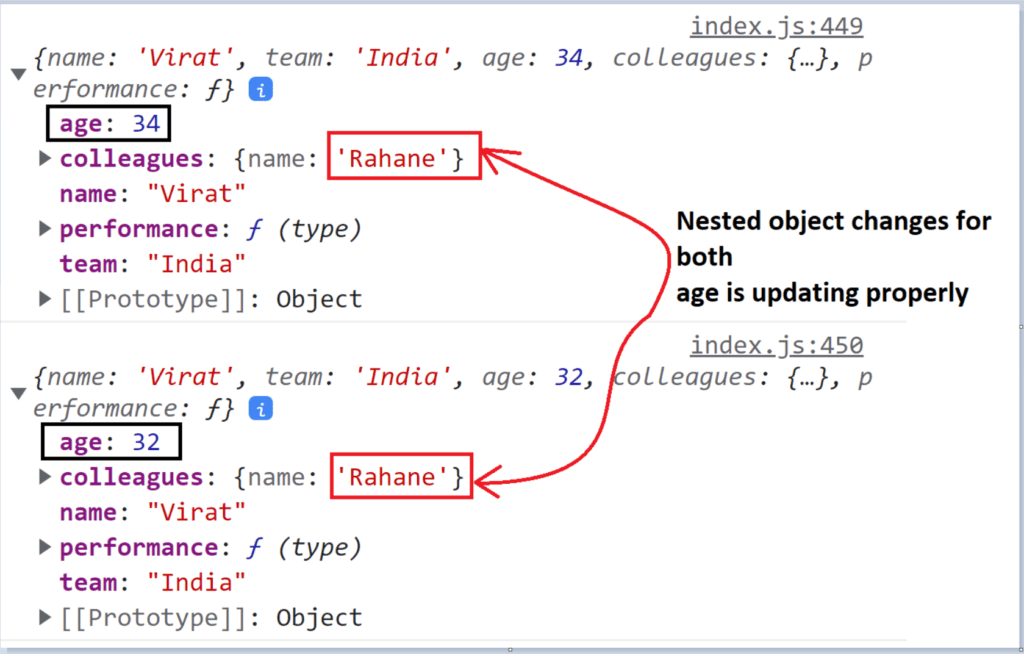
Attempt 5 - use loadash (_.cloneDeep)
It copies properly without any side effects
let finalDeepCopy = _.cloneDeep(cricketer);
finalDeepCopy.colleagues.name = "Rahane";
console.log(finalDeepCopy);
console.log(cricketer);
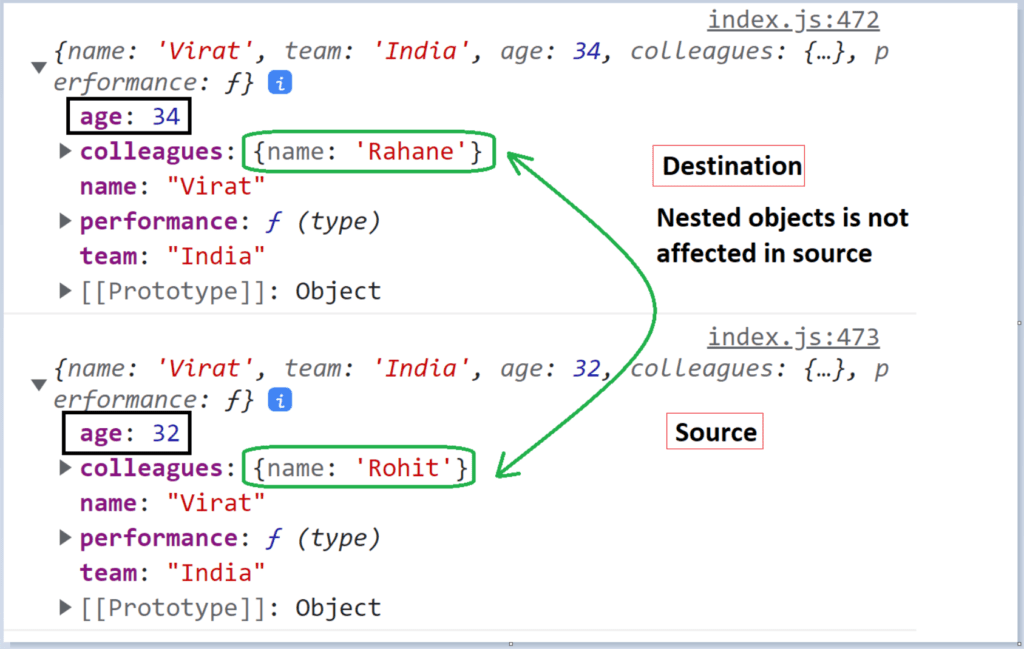