check for undeined/empty/null/false string
let x = null;
x = undefined;
x = "";
x = 0;
x = Number.NaN;
x = false;
console.log(`%c ${x}`, "color:red;font-size:20px");
if (x) {
console.log(`%c I have value`, "color:red;font-size:20px");
} else {
console.log(`%c I am undefined/null/empty`, "color:red;font-size:20px");
}
power of x ^ y
//Short syntax of power of
console.log(2 ** 5); //32
//using Math operation
console.log(Math.pow(2, 5)); //32
use strict
//4.Use Strict
/*
1.cant use a variable without let var or const keyword
2.cant use reserve words as variables
let eval =10
3.cant delete a variable
4.cant delete a function
Best practice always use - 'use strict'
*/
function useStrict() {
"use strict";
result = 10; //this cant be done - rule 1
let eval = 15; //this cant be done - rule 2
delete result; //this cant be done - rule 3
delete useStrict; //this cant be done - rule 4
}
Error?
let @anurag = "anurag";
console.log(@anurag);
//VM3491:1 Uncaught SyntaxError: Invalid or unexpected token
Guess the output
console.log(Number.isNaN(NaN)); //true
//isNaN
//--> checks if its number or not
//-->does type coercion ?? what does it mean ...check below code
console.log(Number.isNaN("2")); //false
Guess the output
//error::->ReferenceError: x is not defined
try {
console.log(x);
} catch (err) {
console.error("error::->" + err);
}
//********************************************
try {
console.log(x);
} catch {
console.error("error");
}
//it gives you the error
//catch no need to take parameter..its optional now
Guess the output on IIFE
//IIFE
(function (a, b) {
console.log((a * b) / b + a);
})(10, 9);
//key points on IIFE
//JS considers above as function expression as it is not starting with function keyword
//func expression means as below
// const x = () =>{} ...
//IIFE is not hoisted by JS engine
10 * 9 =90
90/9 =10
10 + 10 =20
Answer - 20
prototypal inheritence
let Study = function (course, level, isAvailableOnPs) {
this.course = course;
this.level = level;
this.isAvailableOnPs = isAvailableOnPs;
this.courseLevel = function () {
console.log(this.course + " is for " + this.level);
};
};
Study.prototype.IsAvailableOnCoursera = function () {
if (this.isAvailableOnPs) return `${this.course} is available on PluralSight`;
else return `No ${this.course} is not available on PluralSight!!`;
};
let study1 = new Study("Javascript", "Beginners", true);
let study2 = new Study("Advanced Javascript", "Professionals", false);
study1.courseLevel();
study2.courseLevel();
// Javascript is for Beginners
// Advanced Javascript is for Professionals
// ES new concepts is for Proficient
//Prototypal inheritance..
//Every Object has prototype --> where you can add methods and properties to it
//When you create newly created object using prototype-> the newly created object will
//automatically inherit the properties and methods from already existing parent
//It will try to find out in newly created object first--> if it doesnt find ,,will look for parent object
console.log(study1.IsAvailableOnCoursera());
console.log(study2.IsAvailableOnCoursera());
//Javascript is available on PluralSight
//No Advanced Javascript is not available on PluralSight!!
Primitive/Non-Primitive Data Types
key points
⦿ Primitive data type :- string,number,bigint,boolean,undefined,null,symbol
⦿ Non-primitive data type :- object
⦿ Bigint was introduced in ES2020
⦿ Primitive data are those data which are not an object, and has no methods and properties. Also it is immutable.
⦿ Arrays and functions are objects.
⦿ Symbol was introduced in ES2015
⦿ Non-primitive data type :- object
⦿ Bigint was introduced in ES2020
⦿ Primitive data are those data which are not an object, and has no methods and properties. Also it is immutable.
⦿ Arrays and functions are objects.
⦿ Symbol was introduced in ES2015
//*******DataTypes**************
typeof 5 --> number
typeof true --> boolean
typeof 'I am string' --> string
typeof undefined --> undefined
typeof null --> object
typeof Symbol('symbol') --> Symbol
typeof {} --> object
console.log(typeof []); //object (Arrays are objects)
typeof NaN --> Number
//*******Functions**************
const obj = function () {
return 5;
};
obj.hello = "hello";
//Here functions are behaving like objects.you can assign the property
//console.log(obj.hello); // output is hello.
undefined vs null
key points
⦿ undefined == with null returns true
⦿ undefined === with null returns false
⦿ undefined === with null returns false
//****************************************
console.log(`%c ${undefined === null}`, "color:red;font-size:20px");//false
console.log(`%c ${undefined == null}`, "color:red;font-size:20px"); //true
Memory leak
key points
⦿ Runs into infinite loop
⦿ Browser crashes. Kindly see the screenshot below
⦿ Browser crashes. Kindly see the screenshot below
//********************************
let array= [];
for (let i= 15; i > 1; i++) {
array.push(i);
}
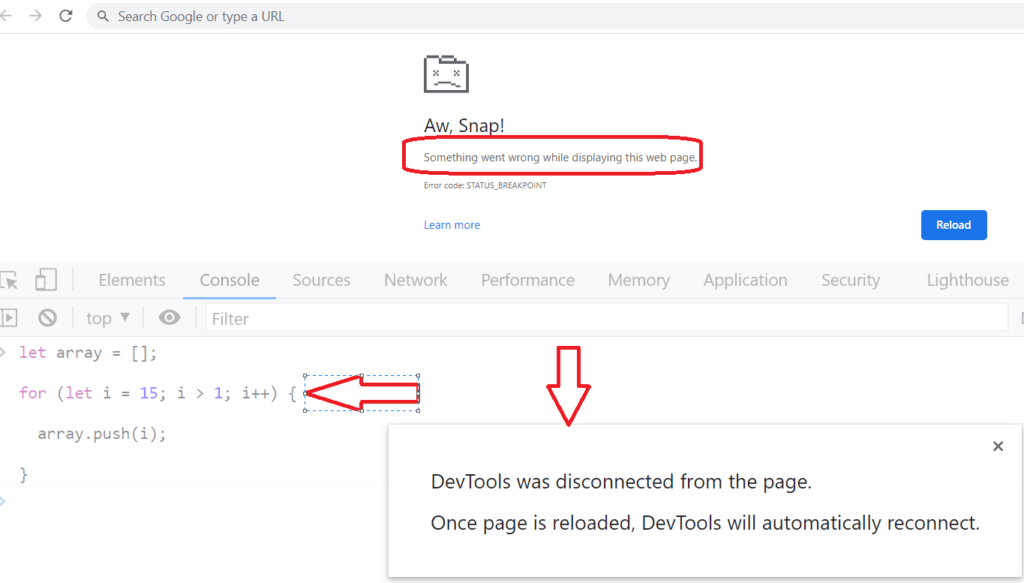
assign values to variable shortcut
//************Assignment**********************
let a, b, c;
//old way
// a = 1;
// b = 2;
// c = 3;
//new way as below
[a, b, c] = [1, 2, 3];
console.log(`%c ${a} ${b} ${c}`, "color:red;font-size:20px");
//output- 1,2,3
Javascript is a dynamic language
key points
⦿ you can assign any data type in javascript, so it is dynamic.
⦿ In the below example, the last value gets updated
⦿ In the below example, the last value gets updated
var a = "anurag";
a = 123;
a = true;
console.log(`%c ${a}`, "color:red;font-size:20px");
//output:- true